Working with text is probably just as common as working with numbers in most programs. Visual Basic refers to text data as strings
and it provides a set of string operators and functions just as it does
for math. Since strings and numbers are very different types of data,
the nature of operators and functions are very different, as shown by Tables 1 and 2.
Table 1. Visual Basic string operators
& (join) | Like (compare) | = (assign) |
Table 2. Visual Basic string functions
Task | Function | Use to |
---|
Compare | Option Compare | Change the string comparison rules |
| Instr | Find one string inside of another |
| StrComp | Compare one string to another |
Convert | Asc | Convert a character to its numeric ANSI value |
| Chr | Convert a numeric ANSI value to a character |
| Format | Convert a number or a date to a string using a specific format |
| LCase | Make a string lowercase |
| UCase | Make a string uppercase |
| StrConv | Change the capitalization, locale, or encoding of a string |
| Val | Get the numeric value of a string |
Arrays | Split | Convert a string to a one-dimensional array |
| Join | Convert a one-dimensional array to a string |
Change | Left | Get a number of characters from the left side of the string |
| Len | Get the length of a string |
| LTrim | Remove spaces from the left side of a string |
| LSet | Copy one string to another, left-aligning the result |
| Mid | Get a specified number of characters from within as string |
| Replace | Search and replace words or characters in a string |
| Right | Get a number of characters from the right side of a string |
| RSet | Copy one string to another, right-aligning the result |
| RTrim | Remove spaces from the right side of a string |
| Trim | Remove spaces from the right and left sides of a string |
Repeat | Space | Create a string containing a number of spaces |
| String | Create a string containing a repeating character |
The following sections explain how to use the string functions to perform the major tasks listed in Table 2.
1. Compare Strings
By default, Visual Basic compares strings in a case-sensitive way. That means "Jeff" and "jeff" are not considered the same. You can change that by adding an Option Compare Text statement at the beginning of a module or class, as shown here:
' Ignore case when comparing
strings.
Option Compare Text
Sub CompareStrings( )
' Displays True if Option Compare is Text.
Debug.Print "Jeff" = "jeff"
End Sub
Option Compare applies to all the ways to compare string (=, Like, StrComp)
throughout the module or class. You can achieve a similar result on a
smaller scale by temporarily converting the strings to upper- or
lowercase before comparing them:
Debug.Print LCase("Jeff") = LCase("jeff")
That approach is actually more common than changing Option Compare since it allows you to use both case-sensitive and case-insensitive comparisons within a class or module.
The Like operator is similar to = in Visual Basic, except it also allows you to match patterns of characters using the comparison characters listed in Table 3.
Table 3. Pattern-matching characters
Use | To match |
---|
? | Any single character |
* | Zero or more characters |
# | Any single digit |
[list] | Any single character in list |
[!list] | Any single character not in list |
For example, the following function returns True if a passed-in argument is formatted as a Social Security number:
Function IsSSN(ssn As String) As Boolean
If ssn Like "###-##-####" Then
IsSSN = True
Else
IsSSN = False
End If
End Function
The Instr
function returns the location of one string within another string. This
is one of the most-used functions in Visual Basic, since it allows you
to break up strings and to do all sorts of search-and-replace tasks.
The StrComp function compares two strings for sorting
. If the first string sorts before the second string, StrComp returns -1; if they sort the same, it returns 0; and if the first string sorts after the second string, StrComp returns 1. The following example demonstrates how to use StrComp to sort an array:
Sub SortArray(arr As Variant, _
Optional compare As VbCompareMethod = vbBinaryCompare)
Dim lb As Integer, ub As Integer, i As Integer, str As String
Dim j As Integer
' If argument is not an array, then exit.
If Not IsArray(arr) Then Exit Sub
lb = LBound(arr)
ub = UBound(arr)
' If only one element, then exit.
If lb = ub Then Exit Sub
For i = lb To ub
str = arr(i)
For j = lb To ub
' Swap values if out of order.
If StrComp(str, arr(j), compare) = -1 Then
str = arr(j)
arr(j) = arr(i)
arr(i) = str
End If
Next
Next
End Sub
There are more efficient sorting routines than the one shown here. I chose this one for its simplicity. |
|
The SortArray procedure lets you specify whether or not to ignore the case of characters when sorting. The default is to use vbBinaryCompare for case-sensitive sorting. You can use the SortArray function to create a function that sorts strings:
Function SortString(str As String, Optional ignorecase = False)
Dim arr As Variant
' Covert the string to an array.
arr = Split(str, " ")
' Sort the array case-sensitive or case-insensitive.
If ignorecase Then
SortArray arr, vbTextCompare
Else
SortArray arr, vbBinaryCompare
End If
' Convert the array back to a string and return it.
SortString = Join(arr, " ")
End Function
To see how these functions work together, step through the following code in the sample worksheet:
Sub DemoSort( )
Dim str As String, arr As Variant
str = "Q z v w p x f g J l h r y D k i e T s u o n M a c b"
' Show case-sensitive sort.
Debug.Print SortString(str, False)
' Show case-insensitive sort.
Debug.Print SortString(str, True)
End Sub
I have an ulterior
motive for showing you these procedures: I'm often asked how you break
tasks into procedures and I think this set of procedures illustrates the
logical division of tasks very well. SortString and SortArray
both make sense as a stand-alone procedure because they might be reused
any number of ways elsewhere in the program. Writing effective,
reusable procedures is one of the key skills that identify you as an
excellent programmer. The best way to learn that skill is by studying
good examples and then practicing on your own!
2. Convert Strings
I touched on two very common conversion functions already: LCase and UCase
convert a string to lower- or uppercase, usually because you want to
ignore case while comparing strings. Your computer can perform these
conversions and comparisons because it actually stores strings as
numbers using something called ANSI character codes
.
The Asc function converts characters to their numeric ANSI character codes; Chr converts those numeric codes back to characters. The following code displays the ANSI character codes in the Immediate window (Figure 3-2):
Sub ShowAnsiCodes( )
Dim i As Integer, str As String
For i = 0 To 255
str = str & i & ": " & Chr(i) & vbTab
If i Mod 10 = 0 Then
Debug.Print str
str = ""
End If
Next
End Sub
Not all character codes have an appearance. Chr(0), Chr(9), Chr(10), and Chr(13) represent the null, tab, line-feed, and carriage-return characters respectively. |
|
Looking at Figure 1,
you can see that you can convert individual characters from upper- to
lowercase by adding 32 or from lower- to uppercase by subtracting 32. UCase and LCase just make those conversions easier.
The StrConv function is related to UCase and LCase.
It can perform the same conversions, plus it can convert the words in a
string to use initial capitalization as is used in proper names:
' Displays St. Thomas Aquinas
Debug.Print StrConv("st. thomas aquinas", vbProperCase)
StrConv
also converts strings to or from other encodings or locales. Those are
pretty advanced topics and I'm just going to skip them here. |
|
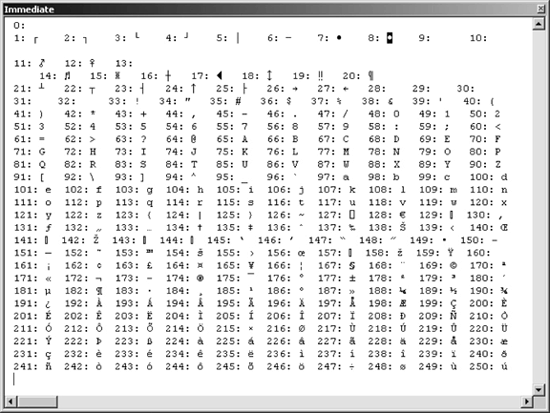
The Format function converts various types of data into strings using predefined or custom formats. The Val
function converts strings containing numeric data back into numeric
data types. This simple example illustrates how these functions work:
Sub ShowFormatVal( )
Dim num As Double, str As String
str = Format(Now, "Short Time")
num = Val(str)
' If the time is 4:31 PM, displays: 16:31 16
Debug.Print str, num
End Sub
You might notice that Val doesn't do anything fancy—it just gets the first part of the string that is numeric and returns it as a number. Val can recognize a few special strings as numbers. For instance, it interprets &HFF as the hexadecimal (base 16) number 255 and &o77 as the octal (base 8) number 63. Format is really more interesting, since it provides useful built-in formats
as listed in Table 4.
Table 4. The Format function's built-in formats
Category | Named format | Converts |
---|
Numeric | General Number | Number to string without thousands separator. This is the default. |
| Currency | Number
to string using the decimal, separator, and currency characters that
are appropriate for the locale. Negative values are enclosed in
parentheses. |
| Fixed | Number to string with at least one digit to the left of the decimal and two digits to the right of the decimal. |
| Standard | Same as Fixed but includes a thousands separator. |
| Percent | Multiplies number by 100 and includes at least two digits to the right of the decimal. |
| Scientific | Number to string using standard scientific notation. |
| Yes/No | Zero to No, nonzero to Yes. |
| True/False | Zero to False, nonzero to True. |
| On/Off | Zero to Off, nonzero to On. |
Date/Time | General Date | Number or date to string using MM/DD/YY HH:MM:SS format. Omits time if number is a whole number. |
| Long Date | Number or date to string using your system's long date format. |
| Medium Date | Number or date to string using your system's medium date format. |
| Short Date | Number or date to string using your system's short date format. |
| Long Time | Number or date to string using your system's long time format. Includes hours, minutes, and seconds. |
| Medium Time | Number or date to string in 12-hour time format. Includes hours, minutes, and AM/PM designator. |
| Short Time | Number or date to string in 24-hour time format. Includes hours and minutes. |
If the built-in formats don't give you what you need, you can build your own format strings using the Format function's formatting codes
listed in Table 5.
Table 5. The Format function's formatting codes
Category | Code | Use to |
---|
String | @ | Include a character or space if there is no character in this position (creates fill spaces for columns). |
| & | Include a character (no fill). |
| < | Force lowercase. |
| > | Force uppercase. |
| ! | Right-align string (default is left-align). |
| \ | Include characters that otherwise have special meaning in the format string (e.g., use \@ to include the @ character). |
| ""literal"" | Include literal characters in a format string. |
Numeric | None | Include number with no special formatting. |
| 0 | Include a digit or zero if there is no digit in this position (creates zero fill). |
| # | Include a digit (no fill). |
| . | Include decimal placeholder. |
| % | Convert number to percentage and include % sign. |
| , | Include a thousands separator. |
| E,-E+, e-,e+ | Convert to scientific notation. |
| −, +, $, ( ) | Include these literal characters (no double quotes or \ is required to include these characters in numeric strings). |
Date/Time | : | Include time separator. |
| / | Include date separator. |
| c | Same as General Date. |
| d, dd | Include day of month as digit. |
| ddd | Include day of week as an abbreviation. |
| dddd | Include full day of week. |
| ddddd | Same as Short Date. |
| dddddd | Same as Long Date. |
| aaaa | Include localized name of the weekday. |
| w | Include day of week as a number (1 to 7). |
| ww | Include week or year as number. |
| m, mm | Include month as number. (Exception: includes minutes if it follows the hour, e.g., hh:mm.) |
| mmm | Include month as an abbreviation. |
| mmmm | Include full month name. |
| oooo | Include full, localized month name. |
| q | Include quarter of year (1 to 4). |
| y | Include day of year (1 to 366). |
| yy, yyyy | Include year as digit. |
| h, hh | Include hour. |
| n, nn | Include minute (or you can use m, mm if following hour). |
| s, ss | Include second. |
| ttttt | Same as Long Time. |
| AM/PM, am/pm, A/P, a/p | Use 12-hour time and include the specified meridian designator. |
| AMPM, ampm | Use 12-hour time and include the system meridian designator. |
Some of the format codes in Tables 4 and 5
refer to system or localized settings. Those codes allow you to use the
calendar and time features from the user's system, rather than using
the default Visual Basic settings, which are based on the Julian
calendar and English month and weekday names. It's important to be aware
of those settings if your program is for use outside of the
English-speaking world.
You can combine formatting code to produce quite sophisticated results. For example:
Debug.Print Format(Now, """Today is ""dddd, mmmm d, yyyy" & _
""" the ""y""th day of the year. The time is now"" ttttt.")
displays this result:
Today is Thursday, June 17, 2004 the 169th day of the year. The time is now
10:28:22 AM.
3. Change Strings
A lot of programming tasks involve getting or changing
parts of a string. For simple replacement tasks, use the Replace method as shown here:
Sub DemoSearchAndReplace( )
Dim str As String
str = "this is some text and some more text"
str = Replace(str, "some", "different")
Debug.Print str
End Sub
The preceding code replaces all instances of some with different in a case-sensitive way. Replace
also provides option for replacing a certain number of occurrences,
starting at a specific position within the string and doing
case-insensitive searches. Replace also replaces one string with another regardless of their length. If the strings are the same length, you could use the Mid statement to change the source string, instead:
Mid(str, InStr(1, str, "text")) = "word"
Debug.Print str
' Displays: this is different word and different more text
The Mid statement is unusual in that it receives an assignment—in this case the replacement string "word". Since Mid
can't make strings longer or shorter, it is mainly useful for modifying
string data that is in a fixed-width format or for replacing single
characters, such as punctuation.
Visual Basic also provides a set of functions to remove leading, trailing, or leading and trailing whitespace characters from a string: LTrim, RTrim, and Trim. Excel does Visual Basic one better by adding the Trim
worksheet function, which removes repeated internal spaces as well. The
following code demonstrates each of the different trim functions:
Sub DemoTrims( )
Dim str As String
str = " this is a string to trim. "
Debug.Print "LTrim:", ">"; LTrim(str); "<"
Debug.Print "RTrim:", ">"; RTrim(str); "<"
Debug.Print "Trim:", ">"; Trim(str); "<"
Debug.Print "Excel Trim:", ">"; _
WorksheetFunction.Trim(str); "<"
End Sub
The preceding code produces the following output in the Immediate window:
LTrim: >this is a string to trim. <
RTrim: > this is a string to trim.<
Trim: >this is a string to trim.<
Excel Trim: >this is a string to trim.<
4. Repeat Characters
Finally, Visual Basic includes a couple of simple functions that create strings of repeated characters
. The Space function returns a string containing spaces, and the String function returns a string containing a repeated character. Those functions are sometimes used in combination with Chr when creating reports or drawing text borders as shown here:
' Draws a little box in the Immediate window.
Sub DrawBox( )
Debug.Print Chr(1) & String(20, Chr(6)) & Chr(2)
Debug.Print Chr(5) & Space(20) & Chr(5)
Debug.Print Chr(5) & Space(20) & Chr(5)
Debug.Print Chr(5) & Space(20) & Chr(5)
Debug.Print Chr(3) & String(20, Chr(6)) & Chr(4)
End Sub